XmlRpcClientS60

Join this project:
To join this project, please contact the project administrators of this project, as shown on the project summary page.
Get the source code:
Source code for this project may be available as downloads or through one of the SCM repositories used by the project, as accessible from the project develop page.

2 XmlRpcClientS60 implementation
2.1 Class structure
2.2 HTTP connections
2.3 Representation of the XML-RPC request
2.4 Representation of the XML-RPC response
3 Developing XML-RPC Client Applications
3.1 Sending request to the server
3.2 Getting response from the server
3.2.1 Handling XML-RPC fault messages
3.3 Dealing with errors
4 Summary
This article describes how to make procedure calls over the Internet using XmlRpcClient library on Symbian OS. Information included in this document refers to XML-RPC protocol. XML-RPC is lightweight protocol for a remote procedure calls. It uses XML to encode its calls and HTTP as a transport mechanism. More information about XML-RPC can be found in document “XML-RPC protocol. Requesting remote services” available as PDF on the right side of this page.
2 XmlRpcClientS60 implementationThe library consists of the following main files:
File name | Description |
---|---|
xmlrpcclient.h | This file contains definition of a class CXmlRpcClient that represents a connection to an XML-RPC server. |
mxmlrpcclientobserver.h | Callback interface to implement in application to be reported by CXmlRpcClient object about response, status, fault etc. |
xmlrpchandler.h | This file contains definition of a class CXmlRpcHanlder that parse XML-RPC messages returned from server and interface MXmlRpcHandlerObserver which CXmlRpcClient is implementing to be notified when parse is finished. |
xmlrpcrequest.h | This file contains definition of a class that represents XML-RPC request. |
xmlrpcresponse.h | This file contains definition of a class that represents XML-RPC response. |
xmlrpcvalue.h | This file contains definition of a class that represents XML-RPC method arguments and results. |
xmlrpcutils.h | This file contains definition of a utility class for converting between Unicode strings and UTF8+xml entities. |
xmlrpcclientstringtable.st | This file contains a static string table specific to the XML-RPC protocol being used by this library. |
Two files not included in the above table, but included in the library, xmlrpcclientstringtable.h and xmlrpcclientstringtable.cpp are auto generate from xmlrpcclientstringtable.st by the Symbian tool called stringtable.pl. A string pool is a Symbian mechanism for storing strings in such a way that makes the comparison of strings a very fast operation.
2.1 Class structureAll classes are found on XmlRpc namespace, with CXmlRpcClient being a main class. Figure 1 shows relationships about all the classes.
Figure 1. Simplified UML class diagram.CXmlRpcClient represents a connection to the server. This class is constructed with one argument, a pointer to object of class that implements interface MXmlRpcClientObserver. CXmlRpcClient reports responses from the Server, faults, and statuses about connection through the supplied MXmlRpcClientObserver.
2.2 HTTP connectionsThis library uses the Symbian HTTP client API that offers direct support for HTTP. This API enables applications to communicate with HTTP servers on the Internet. Use of the HTTP client API is encapsulated in CXmlRpcClient class. HTTP is required for exchanging data with the XML-RPC servers. As the Symbian HTTP client API asynchronously sets up a connection and prepares data to send, calling of method CXmlRpcClient::CallL(CXmlRpcRequest& aRequest) is also asynchronous. This has one good reason – it will not block UI. Figure 2 shows sequence diagram how library is making a call to the XML-RPC server and informing user application about response, status or faults. Below steps describes sequence diagram.
1. Creates new CXmlRpcClient object
2. Sets the address of the XML-RPC host
3. Creates new XML-RPC request
4. Asynchronous call. At this point XmlRpcClient library at the separate thread is doing all the things needed to connect to remote server, send request and parse response. User application is not blocked. When message comes from the server or an error was occurred during a call, user application will be informed via appropriate method of interface MXmlRpcClientObserver.
5, 6, 7. Opens an HttpConnection, sends an XML-RPC request to the server
8. HTTP header was received from the server. Informing the user about status. If all okay, status code 200 OK should be returned.
9, 10, 11. Reading HTTP body data from the Server and parsing it.
12. Inform the user application about response from the Server.
13, 14, 15, 16. Closing application and deleting CXmlRpcClient object.
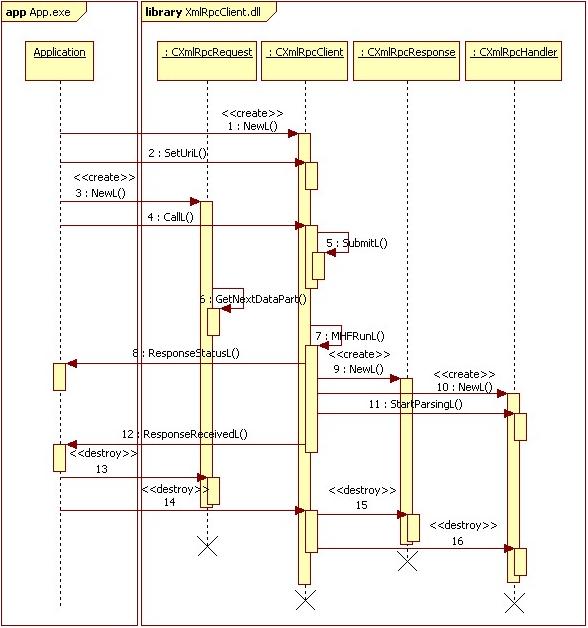
If new call to the Server is needed, user application starts from step 4 or step 3 if another request then previous will be send.
2.3 Representation of the XML-RPC requestXML-RPC request is represented by object of class CXmlRpcRequest. This object is constructed by the user and has all the information about remote method. As the CXmlRpcClient::CallL(CXmlRpcRequest&) is asynchronous call, the user application in some way needs to know to which request server responded. This is done by providing request ID. Request ID is stored only in client side (this is not send to server) and should be unique along the application. When application receive response from the Server, checks for what request this response is by calling RequestId() method on object of class CXmlRpcResponse.
2.4 Representation of the XML-RPC responseResponses from the server are in XML and need to be parsed. A CParser a part of Symbian XML framework is used for parsing XML. A SAX parser in this way is more appropriate as it doesn’t require whole document to be loaded into memory. Parsing is done in class CXmlRpcHandler. This class sets up object of class CXmlRpcResponse which is created by object of class CXmlRpcClient. CXmlRpcResponse contain all the information returned from the Server. Object of class CXmlRpcResponse is create when the data starts flowing from the Server back to the client. Figure 2 shows this on sequence 9. Once this object was constructed it is destroyed only when the user destroy object of the main class CXmlRpcClient. When new data starts flowing from the Server this object is sets with new values (it is not constructing anymore).
According to XML-RPC specification XML-RPC response can contain only one XML-RPC value (object of class CXmlRpcValue). User can obtain pointer to CXmlRpcValue by calling method CXmlRpcResponse::Result().
3 Developing XML-RPC Client ApplicationsWhen developing GUI application on Symbian a typical structure of application looks like below [1]:

Figure 3 shows a minimum number of classes that need to be created to build GUI application on Symbian. The CXmlRpcClient can be placed as a member of class CExampleAppUi. The following example shows simple definition of this class:
class CExampleAppUi : public CAknAppUi, public XmlRpc::MXmlRpcClientObserver { public: // constructor and destructor CExampleAppUi(); virtual ~CExampleAppUi(); void ConstructL(); public: // From CCoeAppUi void HandleCommandL(TInt aCommand); public: // From XmlRpc::MXmlRpcClientObserver void ResponseStatusL(TInt aStatusCode, const TDesC& aStatusText); void ResponseReceivedL(XmlRpc::CXmlRpcResponse& aResponse); void OnError(TInt aErrorCode); public: /** * Call remote methods on the Server. */ void CallL(TInt aRequestId); /** * Cancel an outstanding transaction. */ void CancelCall(); private: void Distance(XmlRpc::CXmlRpcResponse& aResponse); void LatLngPosition(XmlRpc::CXmlRpcResponse& aResponse); private: // AppUi owns the XmlRpcClient engine XmlRpc::CXmlRpcClient* iXmlRpcClient; XmlRpc::CXmlRpcRequest* iXmlRpcRequest; enum TRequestIds { ECalculateDistanceRequestId, EGetLatLngPositionRequestId }; };
Class CExampleAppUi implements also interface MXmlRpcClientObserver so constructing of CXmlRpcClient can be done as follows:
void CExampleAppUi::ConstructL() { iXmlRpcClient = CXmlRpcClient::NewL(*this); // server address and port number _LIT(KXmlRpcServerHost, "http://localhost:8080"); iXmlRpcClient->SetUriL(KXmlRpcServerHost); }
The final implementation of this library is a DLL so user has to change mmp file to link to this library.
/*...*/ LIBRARY XmlRpcClient.lib /*...*/3.1 Sending request to the server
Sending request to the server is done in method CallL of class CExampleAppUi. The following example demonstrates how to create request and call remote server:
void CExampleAppUi::CallL(TInt aRequestId) { delete iXmlRpcRequest; iXmlRpcRequest = NULL; switch (aRequestId) { case ECalculateDistanceRequestId iXmlRpcRequest = CXmlRpcRequest::NewL( _L("server.calculateDistance"), ECalculateDistanceRequestId); break; } if (iXmlRpcRequest) { // call remote method using XmlRpcClient Engine DLL iXmlRpcClient->CallL(*iXmlRpcRequest); } }
First we delete previous request. Then we create new request with the request Id given in the argument. When new XML-RPC request is created user application invoke CallL(CXmlRpcRequest&) on CXmlRpcClient object.
3.2 Getting response from the serverGetting response from the Server is done in two methods. One is ResponseStatusL and second is ResponseReceivedL. In first method as we know from the chapter 2.2 “HTTP connections” library inform user application about the HTTP status we got from server. In the second method, we will get the object of class CXmlRpcResponse which represent XML-RPC response. The following example demonstrates how to get response from the remote server:
void CExampleAppUi::ResponseStatusL(TInt aStatusCode, const TDesC& aStatusText) { iMainContainerView->RemoveXmlRpcCallWaitDialogL(); // XmlRpc HTTP response should always have status code 200. // Other codes are treated as errors. if (aStatusCode != 200) { iMainContainerView->RunGlobalWarningNoteL(&aStatusText); } } void CExampleAppUi::ResponseReceivedL(CXmlRpcResponse& aResponse) { if (aResponse.IsFaultResponse()) { // Handling fault response return; } switch (aResponse.RequestId()) { case ECalculateDistanceRequestId: // Do something with results Distance(aResponse); break; }3.2.1 Handling XML-RPC fault messages
When XML-RPC fault message arrive from the Server to user application, ResponseReceivedL(CXmlRpcResponse& aRespone) is called. Then inside this method user have to check if response is fault response. By calling ErrorCodeL() and ErrorMsg() user can get status code and error message. The following example demonstrates how to check response:
void CExampleAppUi::ResponseReceivedL(CXmlRpcResponse& aResponse) { if (aResponse.IsFaultResponse()) { TBuf<KFaultResponseMaxLength> respBuf; respBuf.Format(KErrFaultResponseFormat, aResponse.ErrorCodeL(), &aResponse.ErrorMsgL()); RunGlobalWarningNoteL(&respBuf); } }3.3 Dealing with errors
If something went wrong, other then XML-RPC fault message or system errors like out-of memory, than library will call OnError(TInt aErrorCode) passing error code. This method is called every time when an error was occurred during the call, a failure occurs if the sever does not respond at all. Also called when error occurred while parsing XML-RPC responses from the server.
This method will not be called when XML-RPC fault messages are received. This type of XML-RPC messages are reported through the ResponseReceivedL() method as mentioned on previous chapter.
This paper has presented an overview of the XmlRpcClient library and demonstrated how to send XML-RPC request to the Server and how to receive and interpret XML-RPC response returned from the Server. This library has been designed to do minimum to communicate with remote servers and call remote methods on that servers. This is full implementation of the XML-RPC protocol and can be used as communication layer in any Symbian applications.